Debug FastAPI with VSCode
To debug a FastAPI
app with VSCode
we are going to go the debug section and create a launch.json
file.
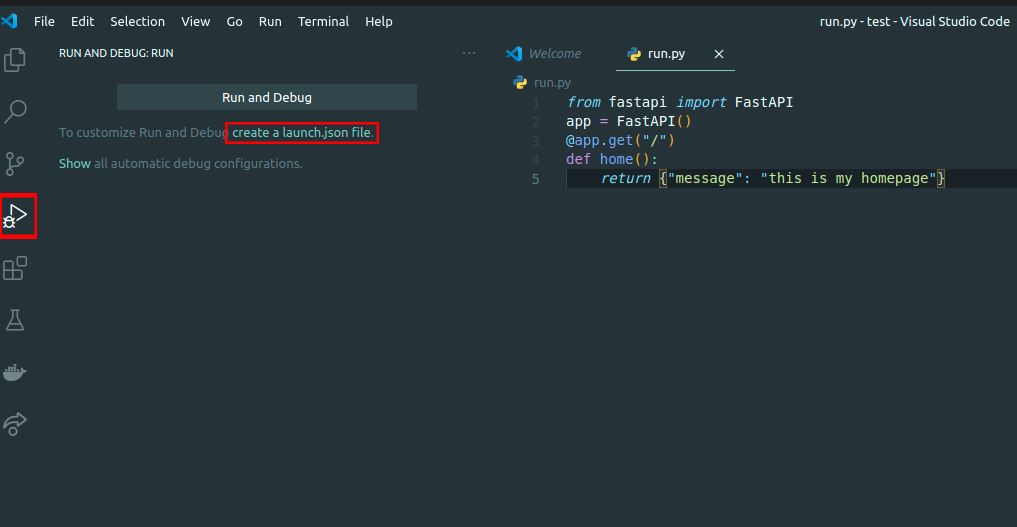
We are going to use the debug template for FastAPI, VSCode
will ask for the name of our file, in my case is run.py
that is located in my root folder.
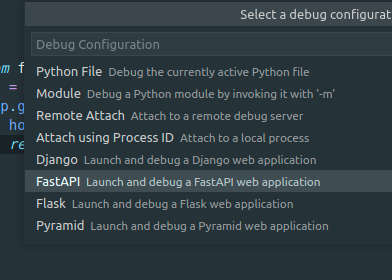
It will generate a launch.json
file in the .vscode
folder
{
"version": "0.2.0",
"configurations": [
{
"name": "Python: FastAPI",
"type": "python",
"request": "launch",
"module": "uvicorn",
"args": [
"run:app"
],
"jinja": true
}
]
}
Make sure that you have your python interpreter set in VSCode
and the libs fastapi
and uvicorn
are installed.
Setting up environments variables in VSCode
To add env vars to the debugging, we need to create env
in the configuration file and set our variables there:
{
...
"configurations": [
{
...,
"env": {
"GOOGLE_CLIENT_ID": "id_from_google",
"SECRET_KEY": "this_is_my_secret_key"
}
}
]
}
Configure FastAPI port in VSCode
We may also want to run the FastAPI app in another port (default port is 8000
), we can set up the port in the args
section:
{
...
"configurations": [
{
...,
"args": {
"--port",
"7000",
"run:app"
}
}
]
}